In this article we look at an MPL3115A2 connected to a Beaglebone first of all lets look at the sensor.
The MPL3115A2 is a compact, piezoresistive, absolute pressure sensor with an I2C digital interface. MPL3115A2 has a wide operating range of 20 kPa to 110 kPa, a range that covers all surface elevations on earth. The MEMS is temperature compensated utilizing an on-chip temperature sensor. The pressure and temperature data is fed into a high resolution ADC to provide fully compensated and digitized outputs for pressure in Pascals and temperature in °C.
This typically comes in a breakout such as the one in the breakout below
Parts Required
Schematic/Connection
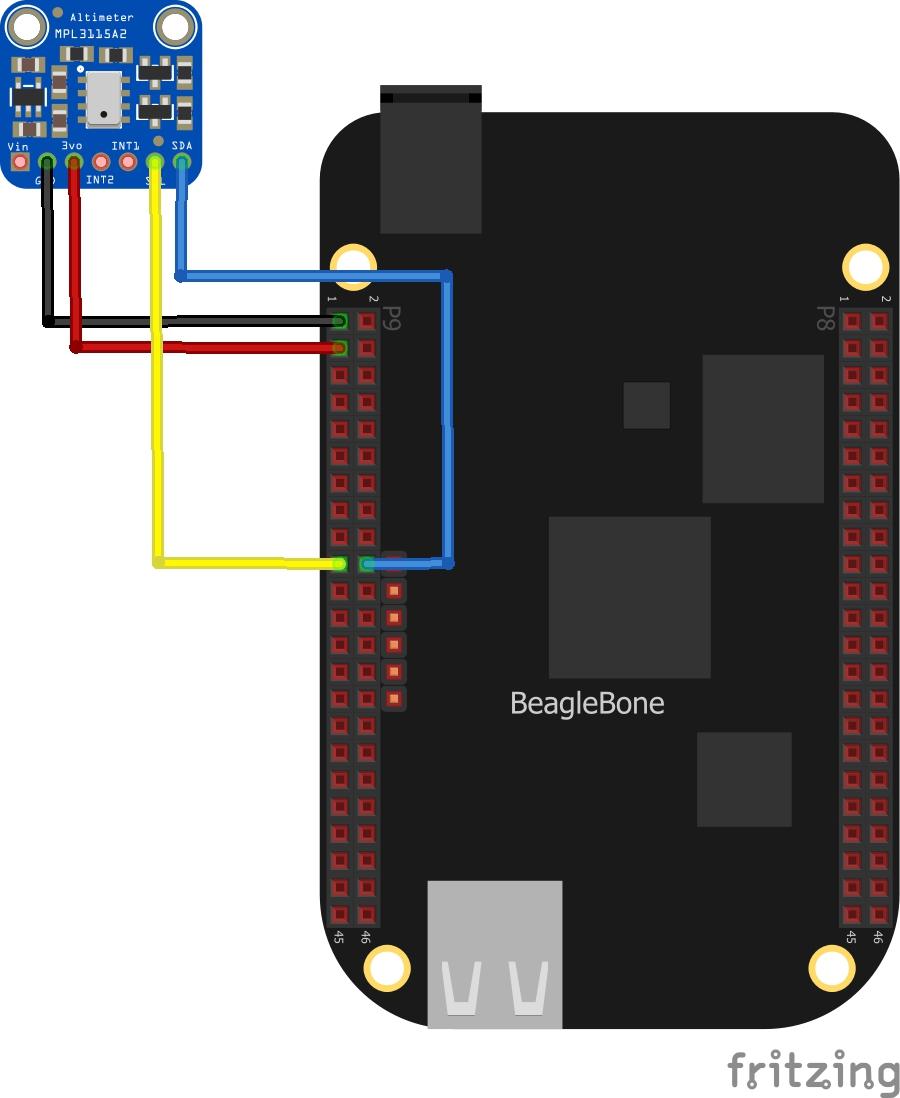
Code Example
This is a controleverything example. This is the C example from https://github.com/ControlEverythingCommunity/MPL3115A2
[codesyntax lang=”cpp”]
#include <stdio.h> #include <stdlib.h> #include <linux/i2c-dev.h> #include <sys/ioctl.h> #include <fcntl.h> void main() { // Create I2C bus int file; char *bus = "/dev/i2c-2"; if((file = open(bus, O_RDWR)) < 0) { printf("Failed to open the bus. \n"); exit(1); } // Get I2C device, MPL3115A2 I2C address is 0x60(96) ioctl(file, I2C_SLAVE, 0x60); // Select control register(0x26) // Active mode, OSR = 128, altimeter mode(0xB9) char config[2] = {0}; config[0] = 0x26; config[1] = 0xB9; write(file, config, 2); // Select data configuration register(0x13) // Data ready event enabled for altitude, pressure, temperature(0x07) config[0] = 0x13; config[1] = 0x07; write(file, config, 2); // Select control register(0x26) // Active mode, OSR = 128, altimeter mode(0xB9) config[0] = 0x26; config[1] = 0xB9; write(file, config, 2); sleep(1); // Read 6 bytes of data from address 0x00(00) // status, tHeight msb1, tHeight msb, tHeight lsb, temp msb, temp lsb char reg[1] = {0x00}; write(file, reg, 1); char data[6] = {0}; if(read(file, data, 6) != 6) { printf("Error : Input/Output error \n"); exit(1); } // Convert the data int tHeight = ((data[1] * 65536) + (data[2] * 256 + (data[3] & 0xF0)) / 16); int temp = ((data[4] * 256) + (data[5] & 0xF0)) / 16; float altitude = tHeight / 16.0; float cTemp = (temp / 16.0); float fTemp = cTemp * 1.8 + 32; // Select control register(0x26) // Active mode, OSR = 128, barometer mode(0x39) config[0] = 0x26; config[1] = 0x39; write(file, config, 2); sleep(1); // Read 4 bytes of data from register(0x00) // status, pres msb1, pres msb, pres lsb reg[0] = 0x00; write(file, reg, 1); read(file, data, 4); // Convert the data to 20-bits int pres = ((data[1] * 65536) + (data[2] * 256 + (data[3] & 0xF0))) / 16; float pressure = (pres / 4.0) / 1000.0; // Output data to screen printf("Pressure : %.2f kPa \n", pressure); printf("Altitude : %.2f m \n", altitude); printf("Temperature in Celsius : %.2f C \n", cTemp); printf("Temperature in Fahrenheit : %.2f F \n", fTemp); exit(0); }
[/codesyntax]
Save this as MPL3115A2.c, I used the Cloud 9 IDE
First of all compile the c program.
$>gcc MPL3115A2.c -o MPL3115A2
Run the c program.
$>./MPL3115A2
Output
After running you should see something like this
Pressure : 99.06 kPa
Altitude : 190.69 m
Temperature in Celsius : 21.56 C
Temperature in Fahrenheit : 70.81 F